Skull and Bones
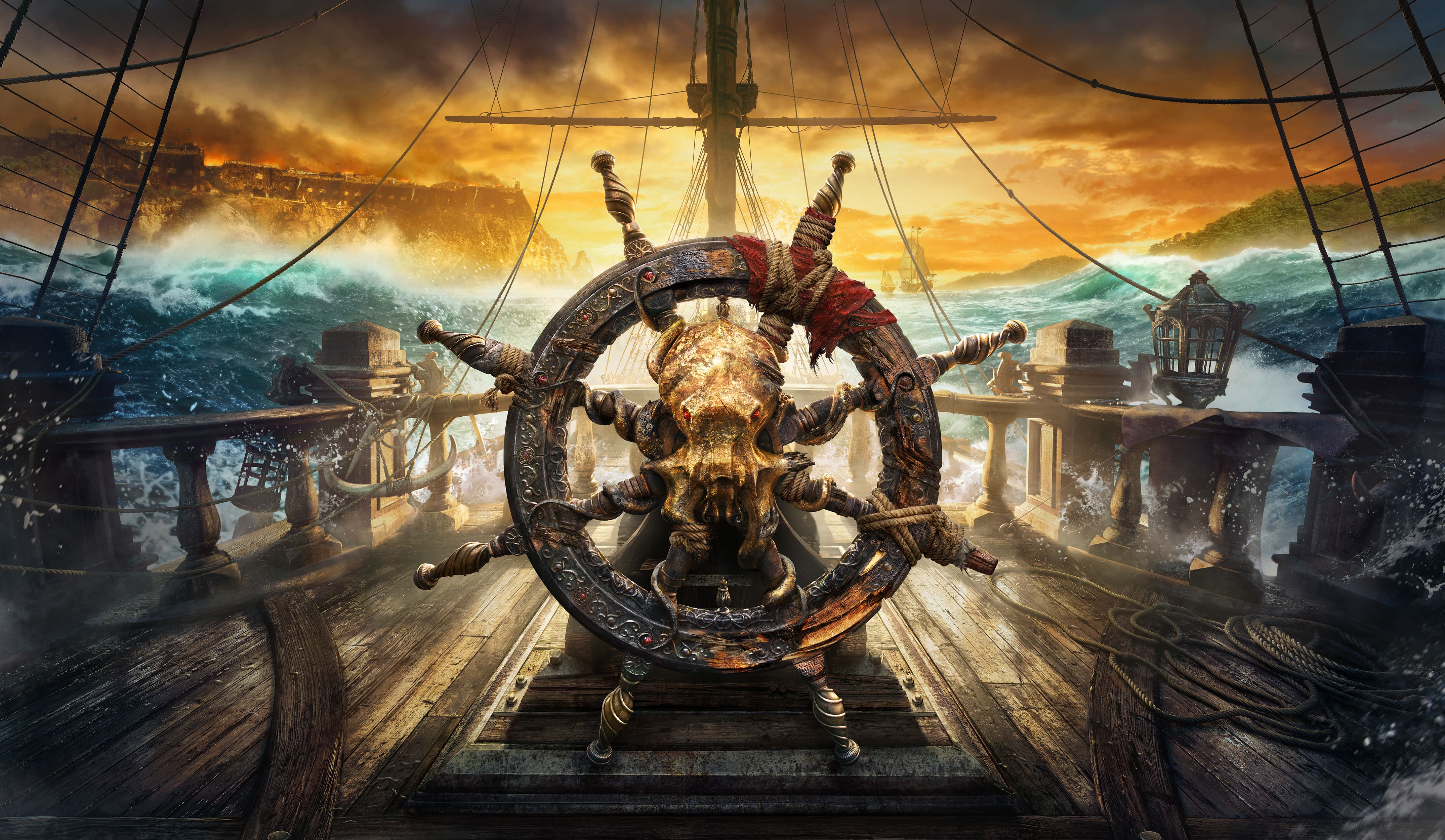
- Environment Query System - Contributed to the implementation of a spatial awareness system used by the AI agents to query the environment in order to find the best position for it to move.
- Archetype Behaviors - Implemented different ship archetypes using Behavior Trees (e.g. guarding ships; ships targeting the player's side; ...).
- Utility AI - Used to decide on the high level behaviors of the agents (e.g. in combat; out of combat; fleeing; ...).
- AI Proxy - Created simple proxy objects representing the agents for when they are out of the player's loading range, allowing them to simulate some simple behaviors and keep some state while very far away from the player, saving computational costs.
- Threat System - Contributed to a system that tracks all entities that are in combat with an agent and how much threat they each pose, allowing to make decisions based on the threat each pose (e.g. attacking the entity that the agent considers more threatening; attacking the least threatening entity first to focus on the others later).
- Attack Ticket System - Contributed to a system that gives out tickets allowing agents to attack other agents or players (e.g. only allow 4 attack tickets to be given to agents targeting the player, so that the player never gets too overwhelmed).
- Dialogue & Story Controller - Implemented a fully event-driven, time-sliced dialogue controller for the game's narrative, supporting concurrent dialogues for both land and sea. This system is able to analyse the state of the game and decide which dialogue scene it should play.
- Sound Matching Lip Sync - Integrated lip syncing technology in the engine, handling the logic for the lip's movements and integrating it into the animation graph.
- Remember Player-Actions System - Implemented a system that keeps track of dozens of player actions, allowing characters to respond to those same actions (e.g. player defeated an enemy with a specific weapon, so some characters might react to that when the player talks to them).
- Dialogue Editor - Supported and extended a tool for the creation of dialogue scenes.
- Created debug tools using ImGui.
- Worked with Technical Animators in the Animation Graph.
- Autonomously drove features forward, actively reaching out to designers and producers to keep things on track.